git initial-commit
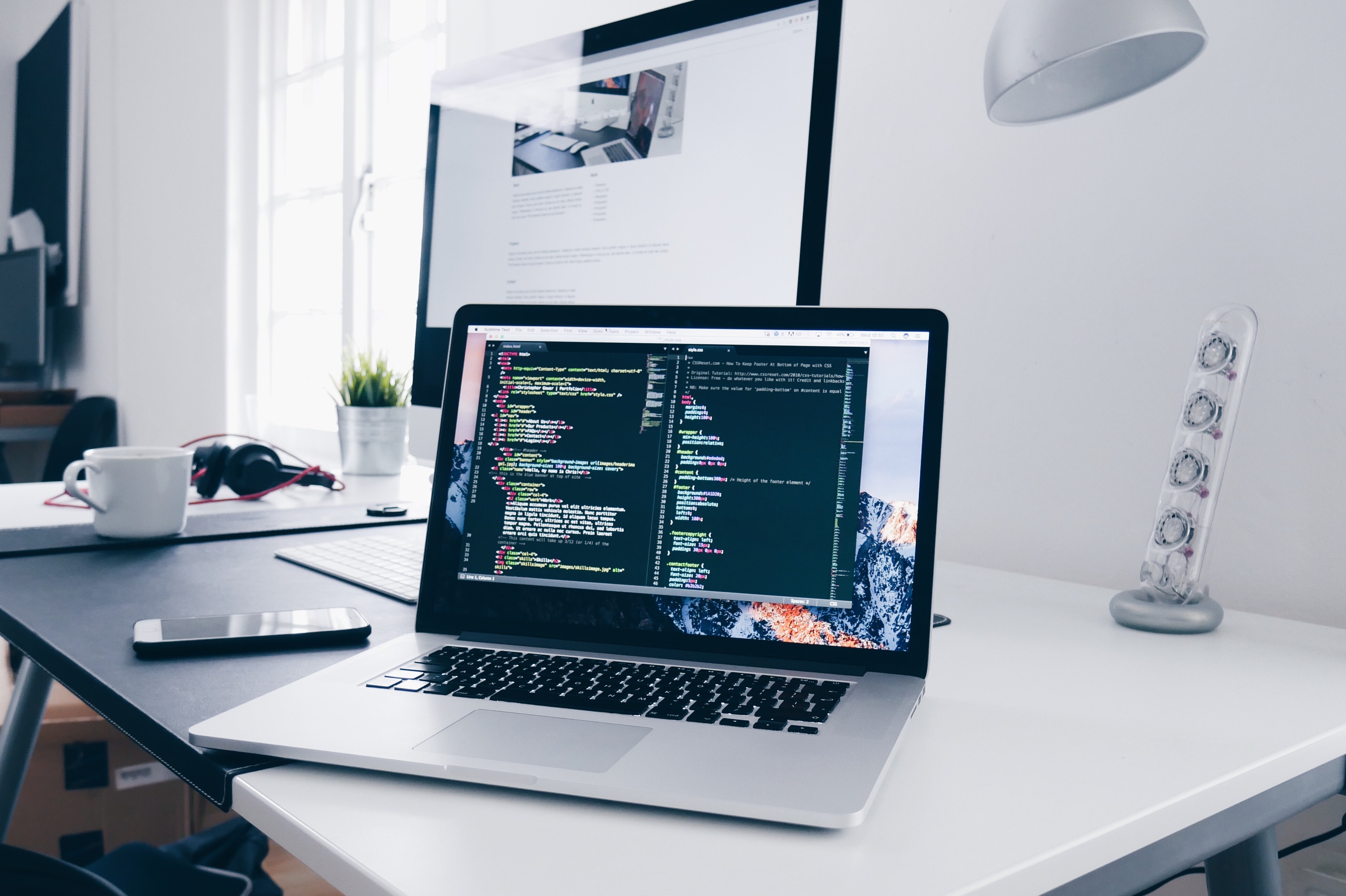
I hate repeating the same manual steps over and over again. I create new git
repositories quite often. I was repeating the same manual steps for each I took some time out to turn it into a repeatable sub-command for git
.
2 minute read
As a conscientious techie I hate repeating the same manual steps over and over
again. Equally so, I find I create new git
repositories quite often. As it
became clear that I was repeating the same manual steps for each I took some
time out to turn it into a repeatable sub-command for git
.
The Command
# file: "~/bin/git-initial-commit"
#!/bin/bash
set -e # exit if any command fails
# if we haven't already initialised, do so, saves an extra manual step
git rev-parse --is-inside-work-tree >/dev/null 2>&1 || git init
# how many commits?
commit_count=$(git rev-list --all --count)
# we want our files to go into the top-level directory ot the project
topdir=$(git rev-parse --show-toplevel)
# grab a .gitignore; assume we have curl
# (currently only writing for ourselves)
if [ -f $topdir/.gitignore ]; then
echo "WARN: $topdir/.gitignore already exists"
else
# now redirects somewhere else
curl -Ls -o $topdir/.gitignore https://gitignore.io/api/git,vim,node,ruby,go
git add $topdir/.gitignore
fi
# drop in a pre-commit config
if [ -f $topdir/.pre-commit-config.yaml ]; then
echo "WARN: $topdir/.pre-commit-config.yaml already exists"
else
# if we have a "preferred" config, use it, otherwise fall back to whatever
# the default is
if [ -f $HOME/.shellrc.d/assets/dot-pre-commit-config.yaml ]; then
echo "INFO: copying preferred .pre-commit-config.yaml"
cp $HOME/.shellrc.d/assets/dot-pre-commit-config.yaml $topdir/.pre-commit-config.yaml
else
echo "INFO: using pre-commit sample-config output"
pre-commit sample-config > $topdir/.pre-commit-config.yaml
fi
git add $topdir/.pre-commit-config.yaml
# make sure we actually use it
pre-commit install
fi
# https://unix.stackexchange.com/a/152554
if [ "${commit_count}" -lt 1 ]; then
git_parms=(-m 'Initial Commit (dotfiles)')
else
git_parms=(-m 'Add dotfiles')
fi
for f in $(git diff --cached --name-only); do
git_parms+=(-m " - add ${f}")
done
git commit "${git_parms[@]}" && git log --oneline -n 1
# vim: filetype=sh
Attributions
- Photo by Christopher Gower on Unsplash