Google Music Cleanup
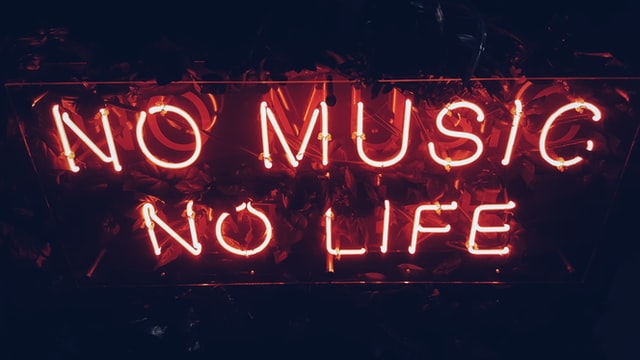
Some days you just need to clean up the mess made by Google Music Manager not recognising pre-existing files.
2 minute read
Although Google Music is on the way out it’s active for a few more months, and if you’re like me you might still have Music manager running, and downloading “updates” to your collection.
Unless it’s just me that Google Music hates you’ll discover that you collection
starts to fill up with MyTrack (1).mp3
and friends.
Being lazy and technical, I have a short script to fix that.
Apologies in advace, but perl
is still my go-to language for quick scripts.
# file: "gm-fixer.pl"
#!/usr/bin/env perl
use strict;
use warnings;
use feature 'say';
use File::Find::Rule;
my @files;
my $dir = $ARGV[0] || die "missing argument";
my $mv_files = $ARGV[1] || 0;
if (! -d $dir) {
die "$dir: not a directory";
}
my @dirs = File::Find::Rule->directory()->maxdepth(1)->mindepth(1)->in($dir);
foreach my $dir (sort @dirs) {
#say "[INFO] checking $dir...";
finder($dir);
}
sub finder {
my $dir = shift;
# find paren-copies
@files = File::Find::Rule->file()
->name( '*([0-9]).mp3' )
->in( $dir );
foreach my $f2 (sort @files) {
my $f = $f2;
$f =~ s{\([1-9]\)}{};
if ($mv_files) {
say "[INFO] moving: '$f2' -> '$f'";
rename($f2, $f) || die "cannot rename: $f2";
}
else {
say "[INFO] found: '$f2'";
}
}
}
Get a feel for the size of the problem:
perl gm-fixer.pl /path/to/root/music/folder
Rename offending files:
perl gm-fixer.pl /path/to/root/music/folder 1